Christian Henschel
Software Engineer
How to pass data between scenes – Static variables
If your game passes the early prototype phase where you just use one scene and build the basic game mechanics, you might come to the point where you start building some test levels to check out some ideas and want to share them with friends, clients (or the world, I dunno).
Let’s say you keep your level selection screen and your game in different scenes (which I prefer to do), how do you pass the selected level id to the game scene where the appropriate data would then be loaded to build the level?
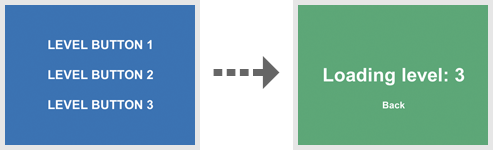
DontDestroyOnLoad
There are different ways to share data between scenes.
You could use a GameObject with a MonoBehaviour script which keeps all attributes you want. You could then call DontDestroyOnLoad on your GameObject to prevent it from getting trashed once the scene is removed.
It could look something like this:
public class MyData : MonoBehaviour { public int currentLevel = 0; void Start() { DontDestroyOnLoad(gameObject); } }
Static attributes
But I think this is rather clunky and there is a much more elegant way to do this by using static class attributes. Static variables aren’t destroyed on scene changes and stay as long as the application is running. I prefer to use a single class called ApplicationModel which keeps things like current level or player data etc. An inheritance of MonoBehaviour isn’t necessary. All the data I’d like to keep available between scenes is placed in there as a static and public attribute.
public class ApplicationModel { static public int currentLevel = 0; }
The currentLevel attribute is updated after the necessary button at the level selection has been clicked. Subsequently the game scene get’s loaded by calling Application.LoadLevel(“MyGame”). After the scene has been loaded the currentLevel value is still available and the appropriate leveldata could be loaded right away.
Levelbutton script example
public class MyButton : MonoBehaviour { public int levelNr = 42; void OnMouseUp() { ApplicationModel.currentLevel = levelNr; Application.LoadLevel("MyGame"); } }
Game script example
public class MyGame : MonoBehaviour { void Start() { int levelNr = ApplicationModel.currentLevel; loadLevelData(levelNr); } void loadLevelData(int nr) { // load level data here } }
Example & Source
A browser demo can be found here
The Unity3D project can be downloaded here
I’ve read your reply on answers.unity and followed here. So you are another blood flash dev now on unity3d heh? Thanks for the tips!
Yep, that’s correct. I wasn’t sure about the future of flash and wanted to try some more tools for game development like Corona & Unity3D. Thanks for leaving a comment.
it works! thank you!
Hey, glad I could help. 🙂
Nice, clean solution!
I do consider all of the concepts you’ve offered to your post.
They’re really convincing and can certainly work. Nonetheless,
the posts are very short for novices. May just you please lengthen them a bit from subsequent time?
Thanks for the post.
Hey, thanks for your comment. I keep that in mind. 😉
I’m not that much of a internet reader to be honest but your sites really nice, keep it up!
I’ll go ahead and bookmark your website to come back later.
All the best
When I was searching for way to pass a parameter, I didn’t like the DontDestroyOnLoad method. Thanks for great tip!
Hey, thanks for leaving a comment. Glad this post is helping someone.
This helped me greatly. Thank you very much sir.
how to make the final score in the game accumulated in unity 3d…\
thanks
Not sure what you mean. You can create a player class with field named score and add points at all necessary positions in your code.
You create an instance of player when you start the game (coming from the menu or levelselection etc.).
public class Player
{
public int score = 0;
public void AddScore(int amount)
{
this.score += amount;
}
}
Just what I was looking for. I knew there had to be some way to do something like this. Thanks for taking the time to blog this. It is a simple and eloquent solution.
Hi Christian,
This ApplicationModel needs to be added as a component to a GameObject in every scene?
In that case, what is the advantage over the DontDestroyOnLoad(gameObject) method?
Regards,
Gerard
Hey Gerard,
static fields are class variables and accessible from everywhere in your code (if made public). You can find more information about this topic here
You access them like this:
Field/Variable
ApplicationModel.myStaticField = “Hello world!”
Method/Function
ApplicationModel.myStaticFunction()
Hope that helps. Thanks for leaving a comment.
Great,
I didn’t know that I could change the values of a static variable, I believed it only serve as a fixed value.
Thanks for the help! 🙂
Cool. I hit that point where I needed to start thinking about multiple levels and throwing data around. This helped a lot (and I need a starting start screen as well!) Thanks for the straight forward post. 🙂
Hey Christopher, thank you. Glad this helped you.
Thank you Christian,
is it your code still current after 3 years? I’ve recently bought a course on Udemy and they use DontDestroyOnLoad method.
Hey Diego, yes this code is still up to date. And it will in be in the future since the solution I explained is Unity independent. You can work with static fields in almost every language. The DontDestroyOnLoad method is more a “beginner” way of doing things since you might not be that experienced with programming in general and static fields can be confusing at first. Also it’s more of a Unity-focused approach which I don’t like.
Hope that helps. Sorry for the late answer. 🙂
Hello, I’m a little bit confused. You just add that class in your scripts folder, not attaching it to any gameobject and it will stay accesible in all your aplication life cycle by any other class?
Yes, if you started programming with Unity you might be confused. C# is more then a fancy scrip-language for Unity. There is a lot more you can do. Maybe you can look here for further information: https://docs.oracle.com/javase/tutorial/java/javaOO/classvars.html (yes, this is for java. But it’s the same in C#). The second one https://www.dotnetperls.com/static is a general introduction to the keyword static. You can use it not only to create static fields but also for static functions. This is useful for the Singleton- or Factory-Pattern for example (https://en.wikipedia.org/wiki/Singleton_pattern, https://en.wikipedia.org/wiki/Factory_method_pattern).
Thank you very much for the example – I kind’a got the meaning; but needed the download to have it make sense.
Hey Jesper, the downloads are fixed and I updated the project files to the current Unity 5.x version.
Why couldn’t i find such a simple and powerful solution in all the tutorials i read/watch about Unity in so many years?
Thanks a lot, this will solve me hours of hard work. 🙂
helpful fresh perspective on use of “dontDestroyOnLoad”; many thanks!