Christian Henschel
Software Engineer
Better Getters & Setters with observers in Swift
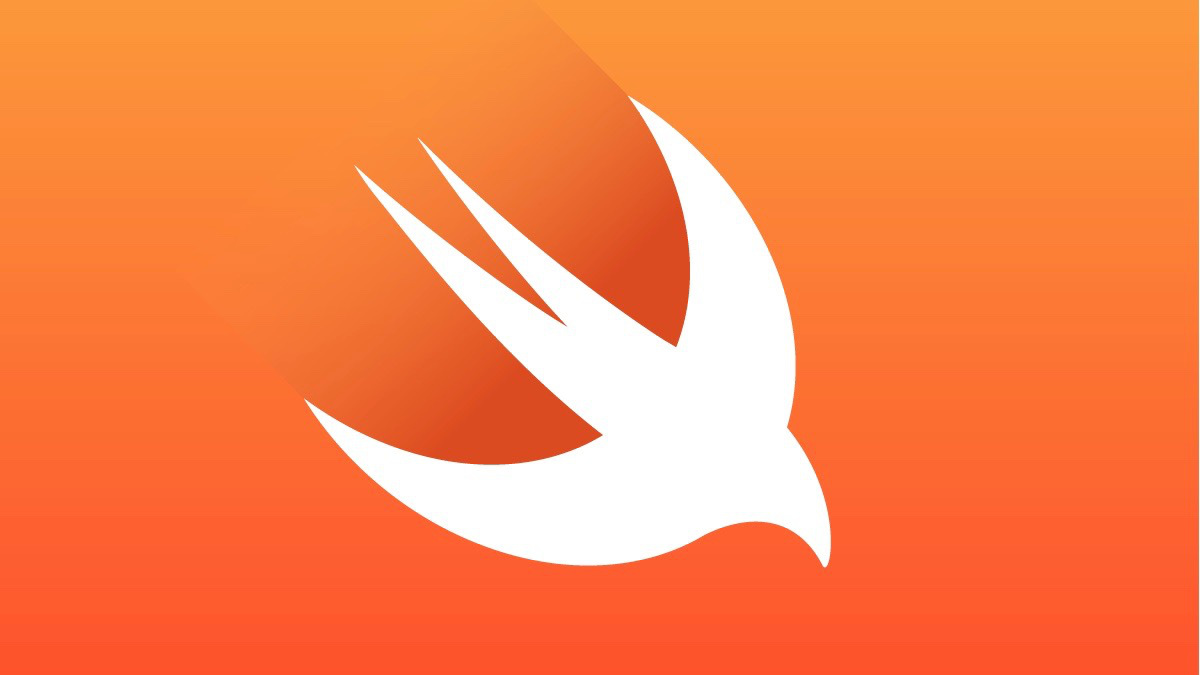
Create Getters & Setters
Better Getters & Setters with observers in Swift… Wow, try to say this three times fast without twisting your tongue.
Ok, whatever.
Using Getter/Setter methods in Swift is pretty straight forward and looks familiar for if you have experience in other languages like Java, C# or ActionScript etc. But an interesting thing is Swifts observer functionality that gives you more control over these methods. But first we look at the standard way we use getters/setters in other programming languages.
In case you ask yourself were the newValue is coming from: The newValue is set by default if no parameter is defined by the Setter method.
// Class definition class MyVector { var x = 0; var y = 0 var halfX: Int { get { return x / 2 } set { x = 2 * newValue } } } // Testing MyVector class getters & setters var v2:MyVector = MyVector() print("V2 => x:\(v2.x), y:\(v2.y), halfX:\(v2.halfX)") v2.halfX = 10; print("V2 => x:\(v2.x), y:\(v2.y), halfX:\(v2.halfX)")
[adinserter block=”10″]
Property observers
Ok, and now the fancy new stuff: Observers allow you to execute code when a value is set via setter method. For this there are the keywords: willSet and didSet. In the example below the Setter is printing a message when it is about to set a new value using the willSet statement. Further the Setter checks if the new value is greater 100 and corrects the value if this is the case. This is done by using the didSet statement.
class MyVector2 { var x: Int = 0 { willSet { print("About to change x to \(newValue)") } didSet { if x > 100 { x = 100 print("X > 100 => set to 100") } } } } var v5 = MyVector5() v5.x = 10 // Output: "About to change x to 10" & "X > 100 => set to 100"